Flutter 组件基础 ——ListView#
ListView 是滚动列表,类似于 iOS 中 ScrollView,可横向、纵向滚动,内容不限。
ListView 的使用#
ListView 的使用很简单,但是需要多多练习;
ListView 的使用,通过设置 children 来实现,children 中的 Item 为 Widget 对象。
纵向滚动#
代码如下:
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'ListView Learn',
home: Scaffold(
appBar: new AppBar(
title: new Text('ListView Widget')
),
body: new ListView(
children: <Widget>[
Container(
height: 50,
color: Colors.orangeAccent,
child: const Center(
child: Text('Entry A'),
),
),
Container(
height: 50,
color: Colors.lightGreenAccent,
child: const Center(
child: Text('Entry B'),
),
),
new ListTile(
leading: new Icon(Icons.access_time),
title: new Text('access_time'),
),
new Image.network(
'https://inews.gtimg.com/newsapp_ls/0/13792660143/0.jpeg')
],
)
)
);
}
}
效果如下:
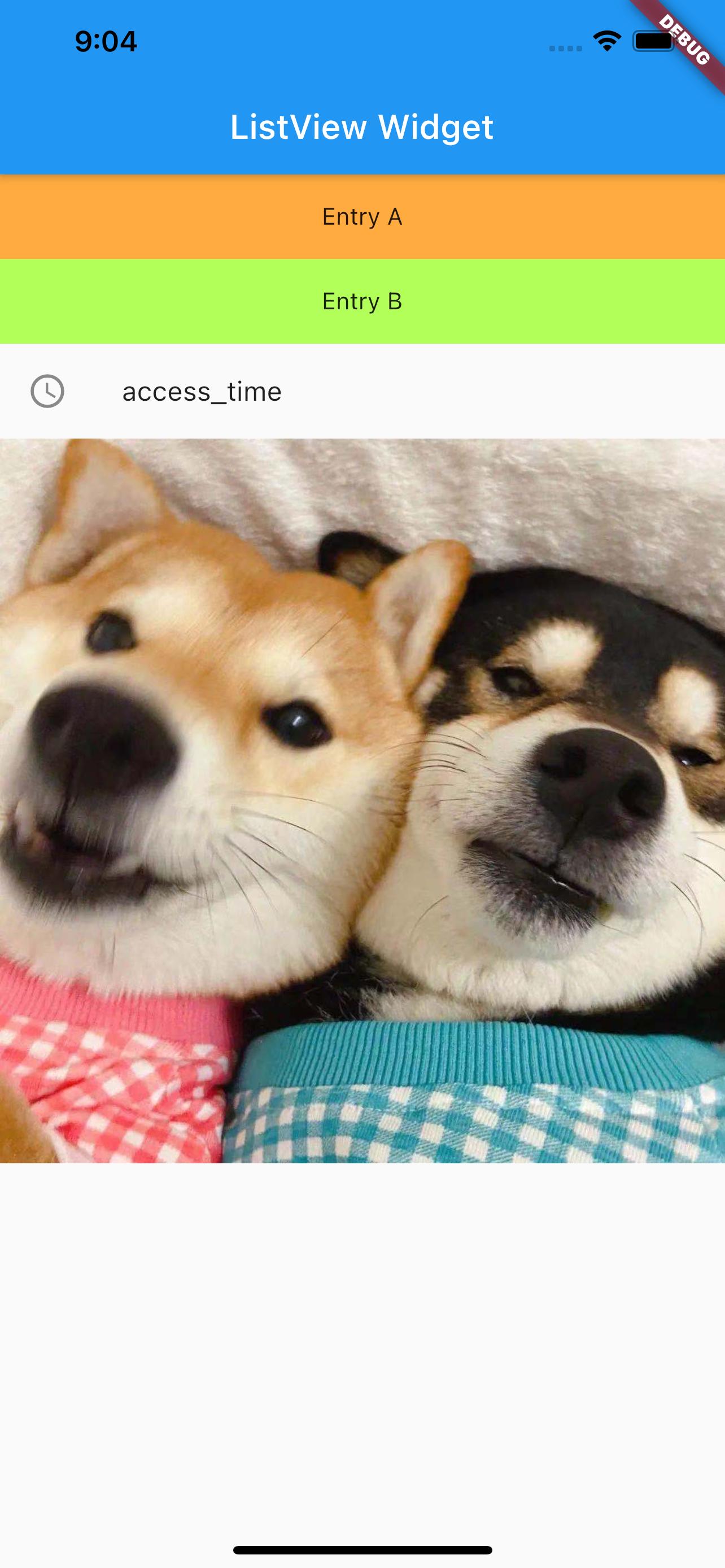
横向滚动#
ListView
的scrollDirection
控制滑动方向
代码如下
class MyList extends StatelessWidget {
@override
Widget build(BuildContext context) {
return ListView(scrollDirection: Axis.horizontal, children: [
new Container(
width: 180.0,
color: Colors.lightBlue,
),
new Container(
width: 180.0,
color: Colors.lightGreen,
),
new Container(
width: 180.0,
color: Colors.orange,
),
new Container(
width: 180.0,
color: Colors.orangeAccent,
)
]);
}
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Text Widget',
home: Scaffold(
body: Center(
child: Container(
height: 200.0,
child: MyList(),
),
),
));
}
}
效果如下:
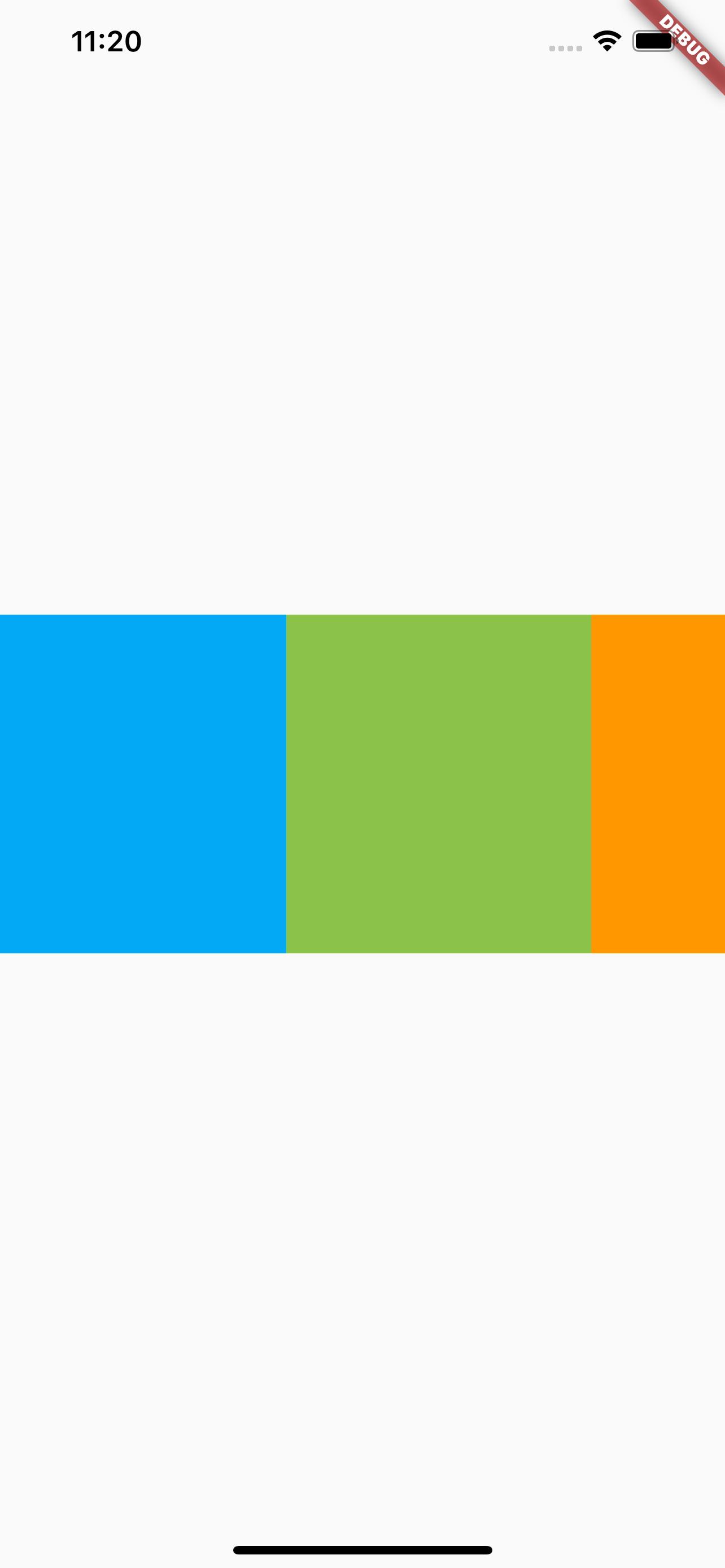
注意写法的不同,在这里自定义了一个MyList
的 Widget,然后在MyApp
中使用MyList
,就避免了在父视图嵌套太多的问题。
动态列表 ListView.builder ()#
使用动态列表需要先来看一下 List 类型,
List 类型
List 是集合类型,声明有几种方式,使用方式可以参考 Swift 中的 Array
var myList = List()
: 非固定长度的数组var myList = List(2)
: 长度为 2 的数组var myList = List<String>()
: 创建一个 String 类型的数组var myList = [1, 2, 3]
: 创建一个 1、2、3 的数组
也可以使用generate
方法来生成 List 元素,比如
new List<String>.generate(1000,, (i) => "Item $i");
动态列表
代码如下:
class MyList extends StatelessWidget {
final List<String> entries = <String>['A', 'B', 'C'];
final List colors = [
Colors.orangeAccent,
Colors.lightBlueAccent,
Colors.cyanAccent
];
@override
Widget build(BuildContext context) {
return ListView.builder(
padding: const EdgeInsets.all(8),
itemCount: entries.length,
itemBuilder: (BuildContext context, int index) {
return Container(
height: 50,
color: colors[index],
child: Center(
child: Text('Entry ${entries[index]}'),
),
);
},
);
}
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'List Build Learn',
home: Scaffold(
appBar: new AppBar(
title: new Text('List Build Learn'),
),
body: Center(
child: Container(
child: MyList(),
),
),
));
}
}
效果如下:
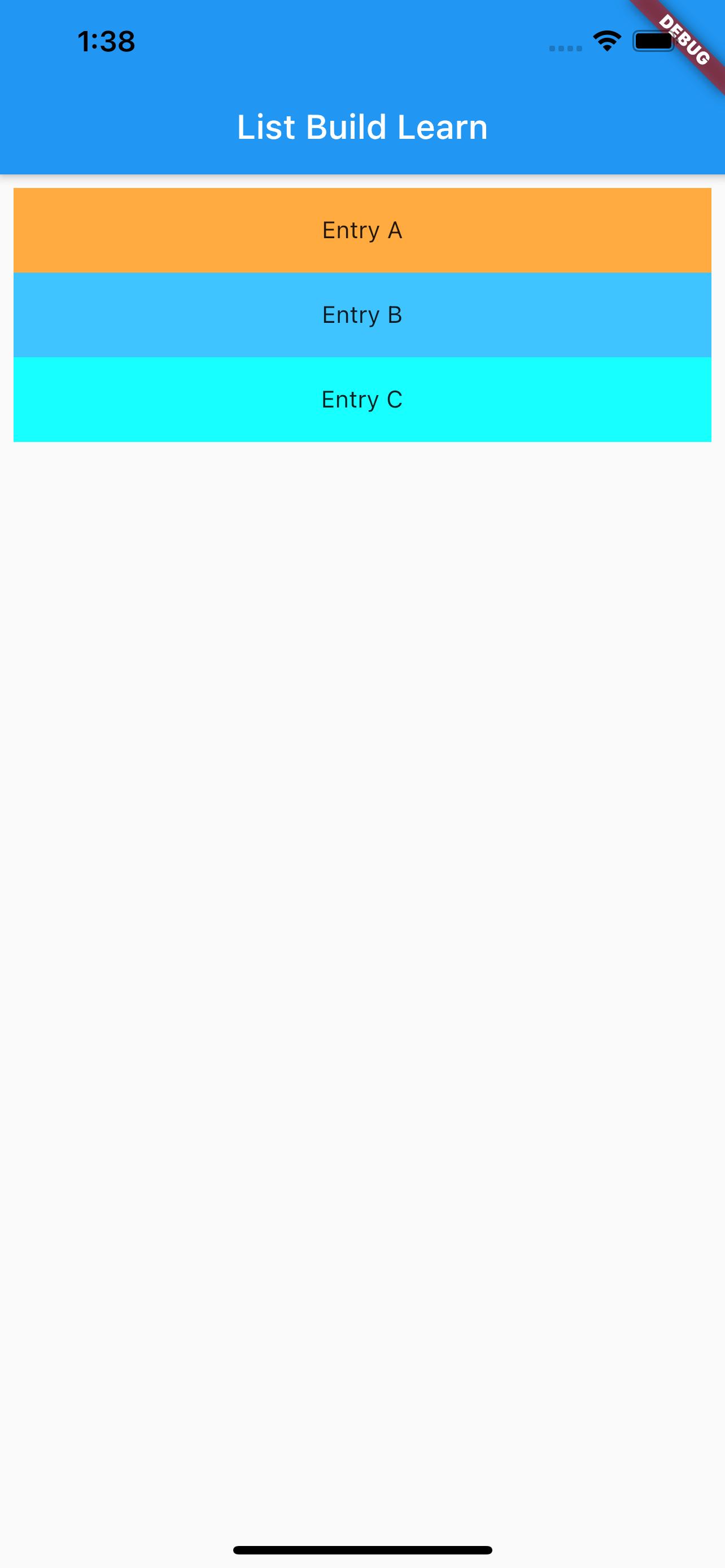