Flutter 元件基礎 ——Container#
Container 是容器元件,類似於 H5 中的
Container 包含屬性#
Container 常用屬性如下:
- Container
- child:子視圖
- alignment:子視圖的對齊方式
- topLeft:頂部左對齊
- topCenter:頂部居中對齊
- topRight:頂部右對齊
- centerLeft:中間左對齊
- center:中間對齊
- centerRight:中間右對齊
- bottomLeft:底部左對齊
- bottomCenter:底部居中對齊
- bottomRight:底部右對齊
- color:背景顏色
- width:寬度
- height:高度
- padding:子視圖距 Container 的邊距
- margin:Container 距父視圖的邊距
- decoration:裝飾
子視圖對齊方式 - alignment#
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Container Learn',
home: Scaffold(
body: Center(
child: Container(
child: Text(
'Alignment center',
style: TextStyle(fontSize: 40.0),
),
alignment: Alignment.center,
width: 500.0,
height: 400.0,
color: Colors.lightBlue,
),
),
),
);
}
}
效果圖如下:
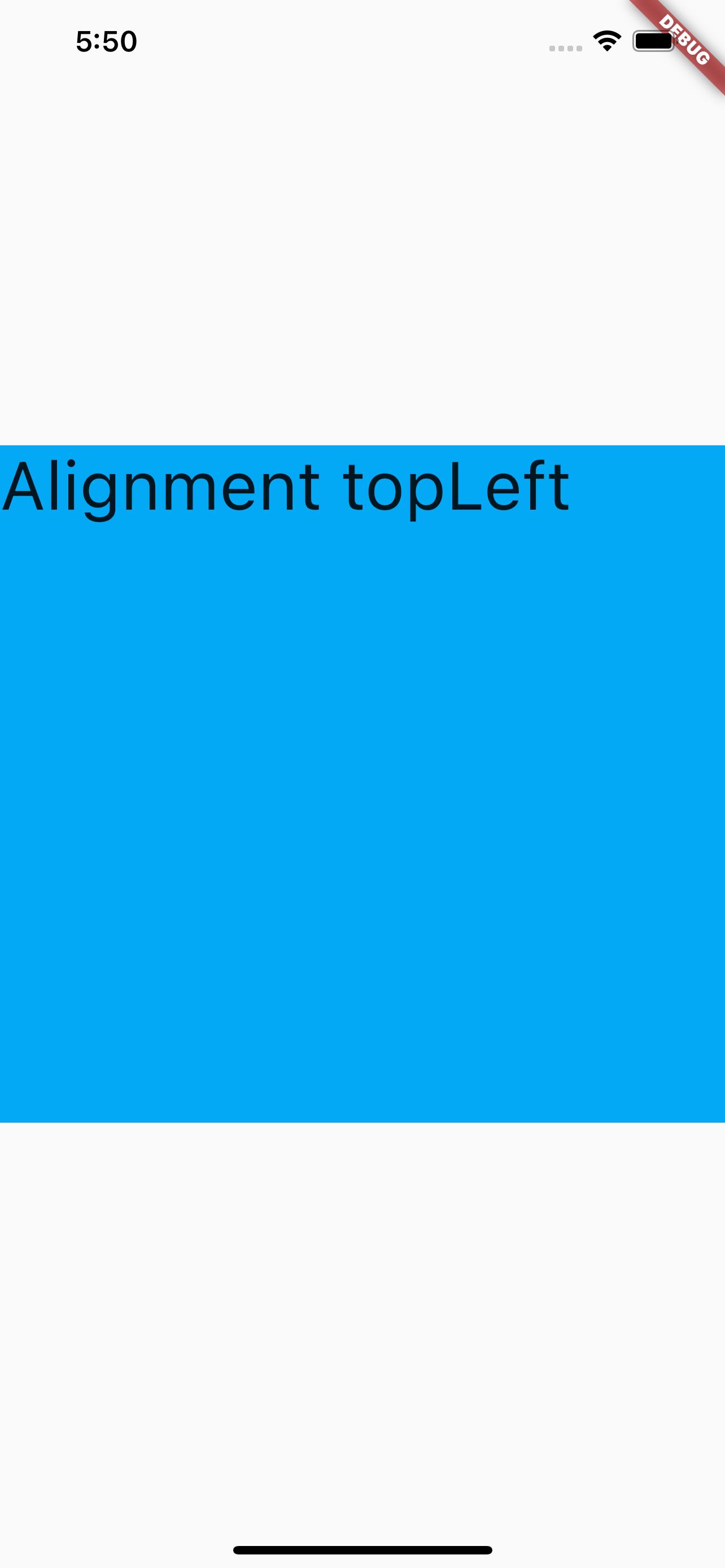
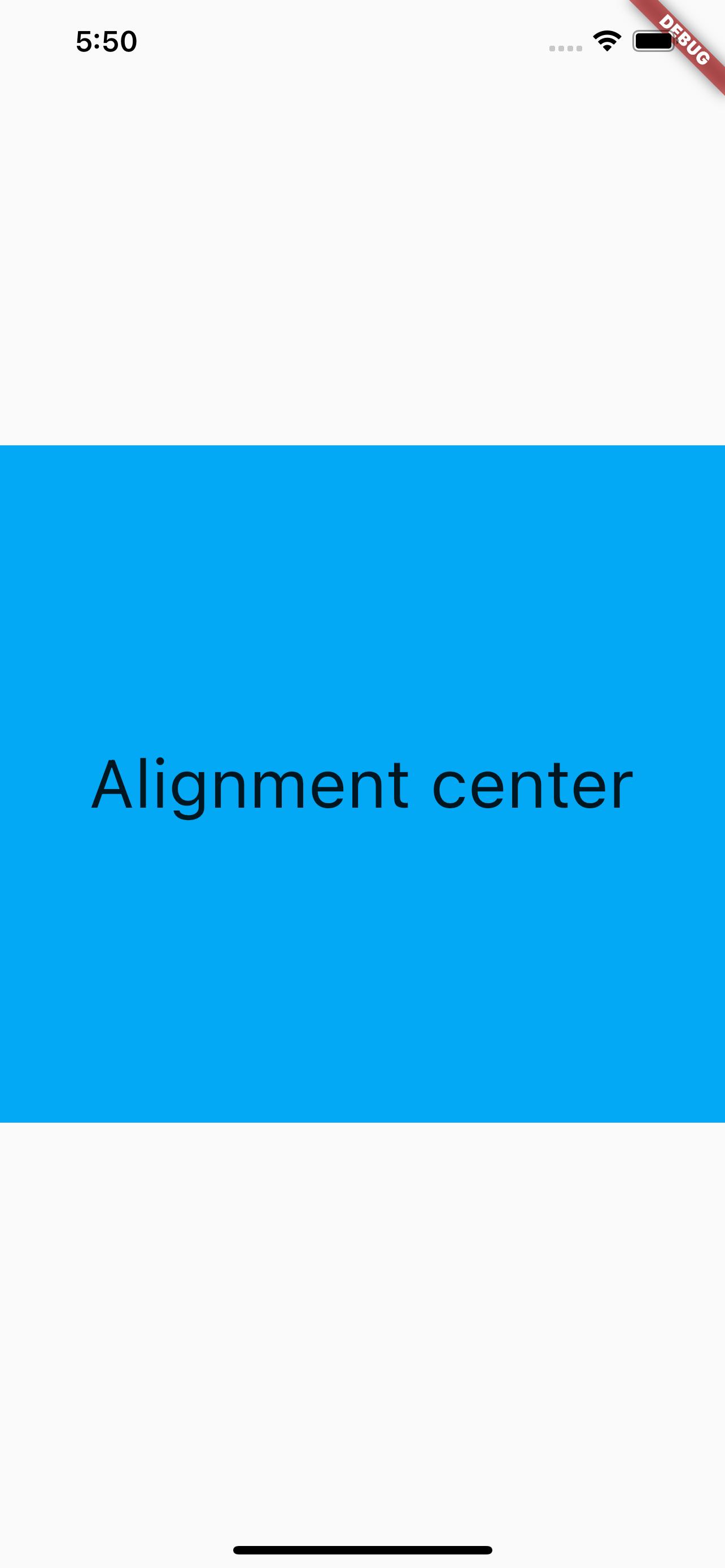
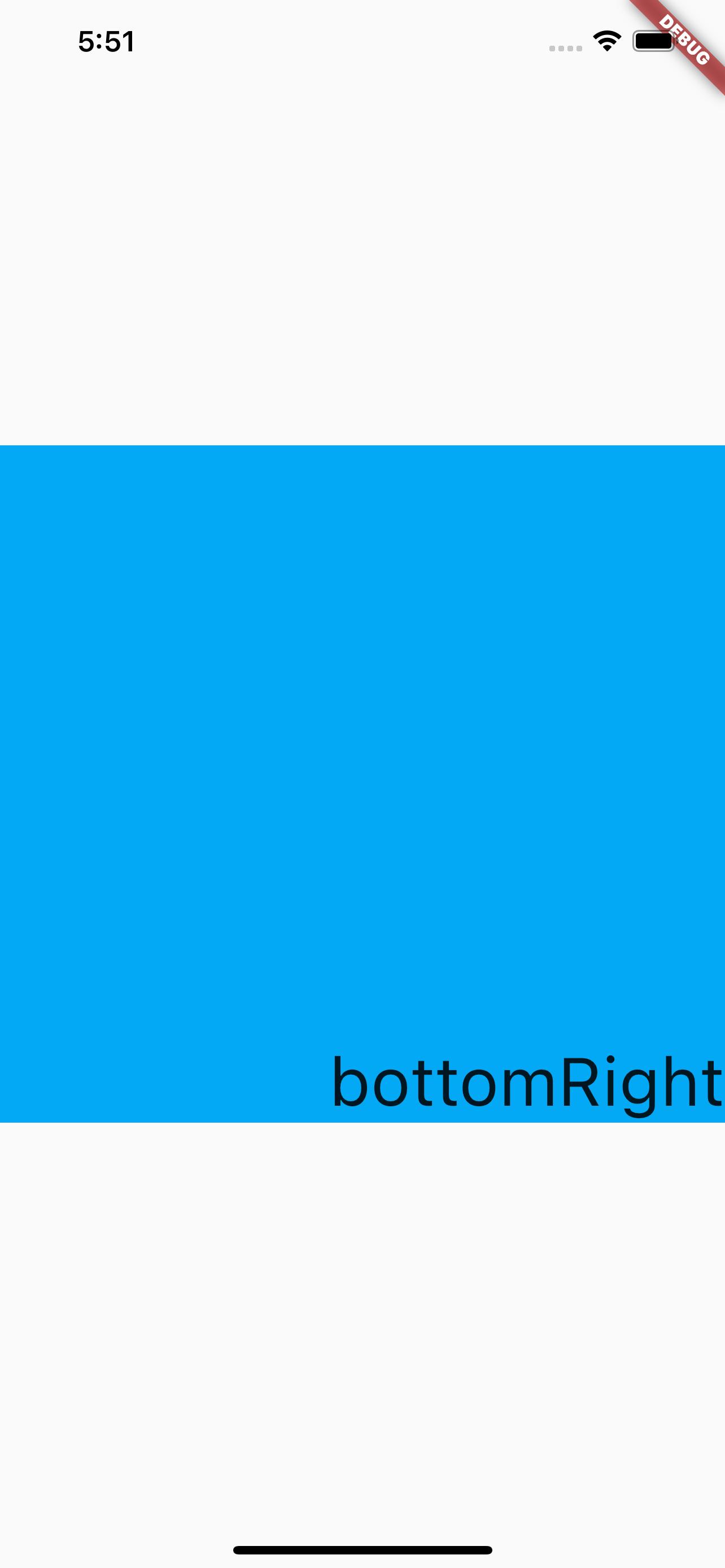
Container 寬、高#
width 和 height 的設定,直接是固定的值。
沒有類似 H5 那種 '100%' 的設定。所以如果想要設定 Container 為螢幕寬高時,可以用以下的方法:
方法一:
import 'dart:ui';
final width = window.physicalSize.width;
final height = window.physicalSize.height;
Container(
color: Colors.red,
width: width,
child: Text("寬度有多寬"),
)
方法二:
Container(
color: Colors.red,
width: double.infinity,
child: Text("寬度有多寬"),
)
子視圖距 Container 的邊距 - padding#
padding 設定的是子視圖,距 Container 的邊距,兩種設定方式,通常有兩種設定方式,EdgeInsets.all
常用於設定所有邊距都一致;EdgeInsets.fromLTRB
用於設定指定邊距(LTRB 對應的 Left、Top、Right、Bottom)。代碼如下:
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Container Learn',
home: Scaffold(
body: Center(
child: Container(
child: Text(
'padding left: 10, top: 20',
style: TextStyle(fontSize: 40.0),
),
alignment: Alignment.topLeft,
width: 500.0,
height: 400.0,
color: Colors.lightBlue,
padding: const EdgeInsets.fromLTRB(10.0, 20.0, 0.0, 0.0),
// padding: const EdgeInsets.all(20),
),
),
),
);
}
}
顯示效果如下:
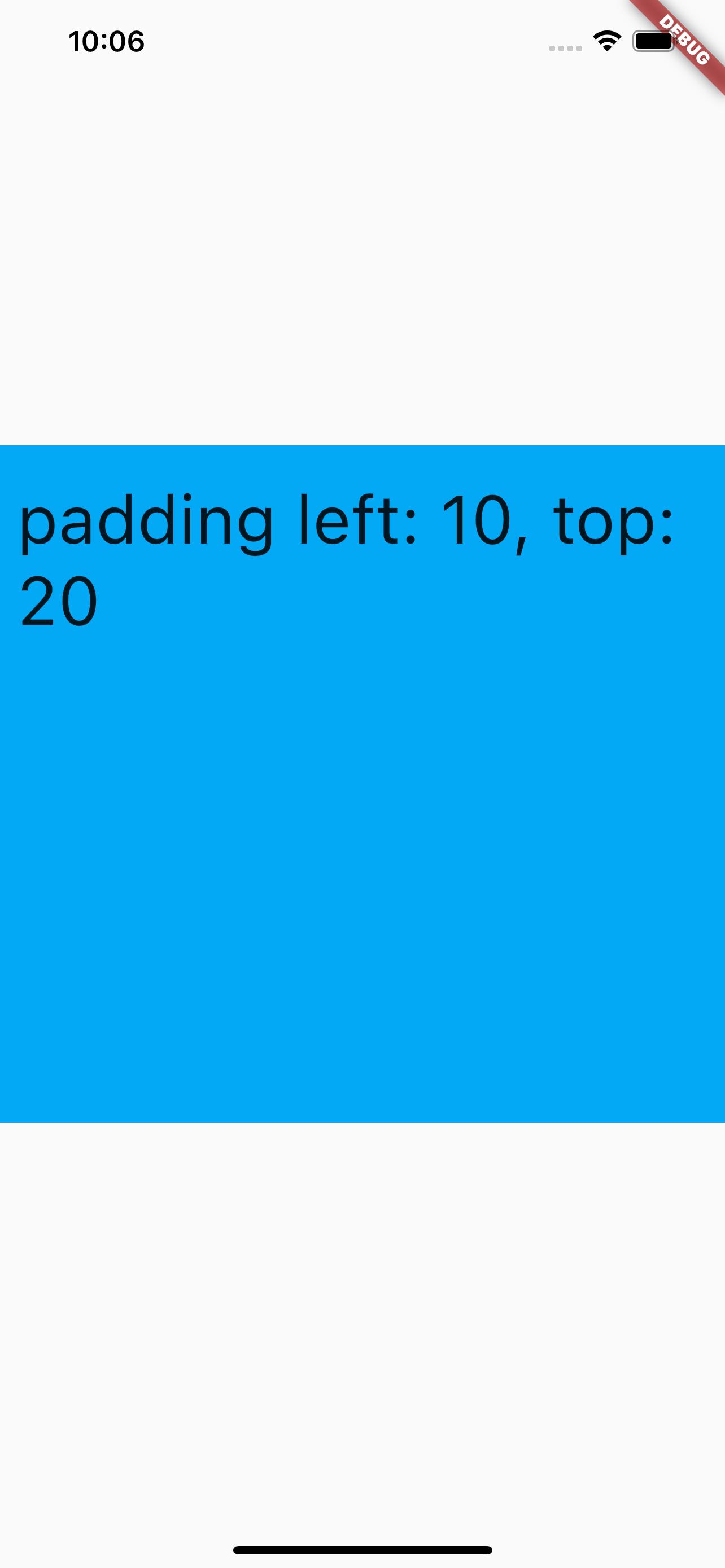
container 距父視圖的邊距 - margin#
margin 的設定和 padding 相同,效果對比,可以先註解 width 和 height,代碼如下:
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Container Learn',
home: Scaffold(
body: Center(
child: Container(
child: Text(
'margin all 30',
style: TextStyle(fontSize: 40.0),
),
alignment: Alignment.topLeft,
// width: 500.0,
// height: 400.0,
color: Colors.lightBlue,
// padding: const EdgeInsets.fromLTRB(10.0, 20.0, 0.0, 0.0),
// padding: const EdgeInsets.all(20),
margin: const EdgeInsets.all(30.0),
),
),
),
);
}
}
效果如下:
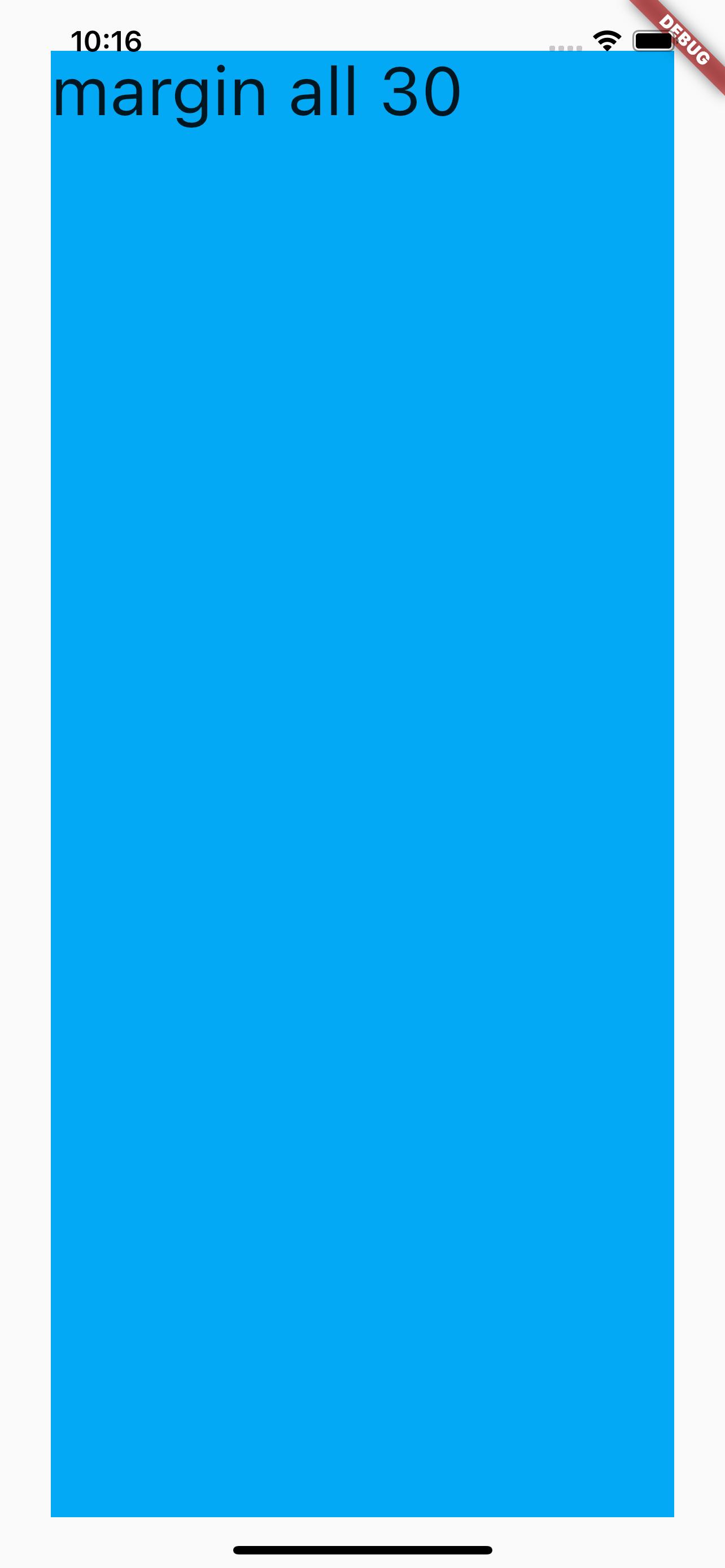
container 的 decoration#
decoration 可用於設定背景色、背景漸變效果、邊框效果等,需要注意 decoration 和 color 不能同時設定,如果需要設定,可以通過在 decoration 中設定 color 來實現,代碼如下:
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Container Learn',
home: Scaffold(
body: Center(
child: Container(
child: Text(
'margin all 30',
style: TextStyle(fontSize: 40.0),
),
alignment: Alignment.topLeft,
width: 500.0,
height: 400,
// color: Colors.lightBlue,
// padding: const EdgeInsets.fromLTRB(10.0, 20.0, 0.0, 0.0),
// padding: const EdgeInsets.all(20),
// margin: const EdgeInsets.all(30.0),
decoration: BoxDecoration(
gradient: const LinearGradient(colors: [
Colors.lightBlue,
Colors.greenAccent,
Colors.purple,
]),
border: Border.all(width: 10.0, color: Colors.red),
color: Colors.lightBlue)),
),
),
);
}
}
效果如下:
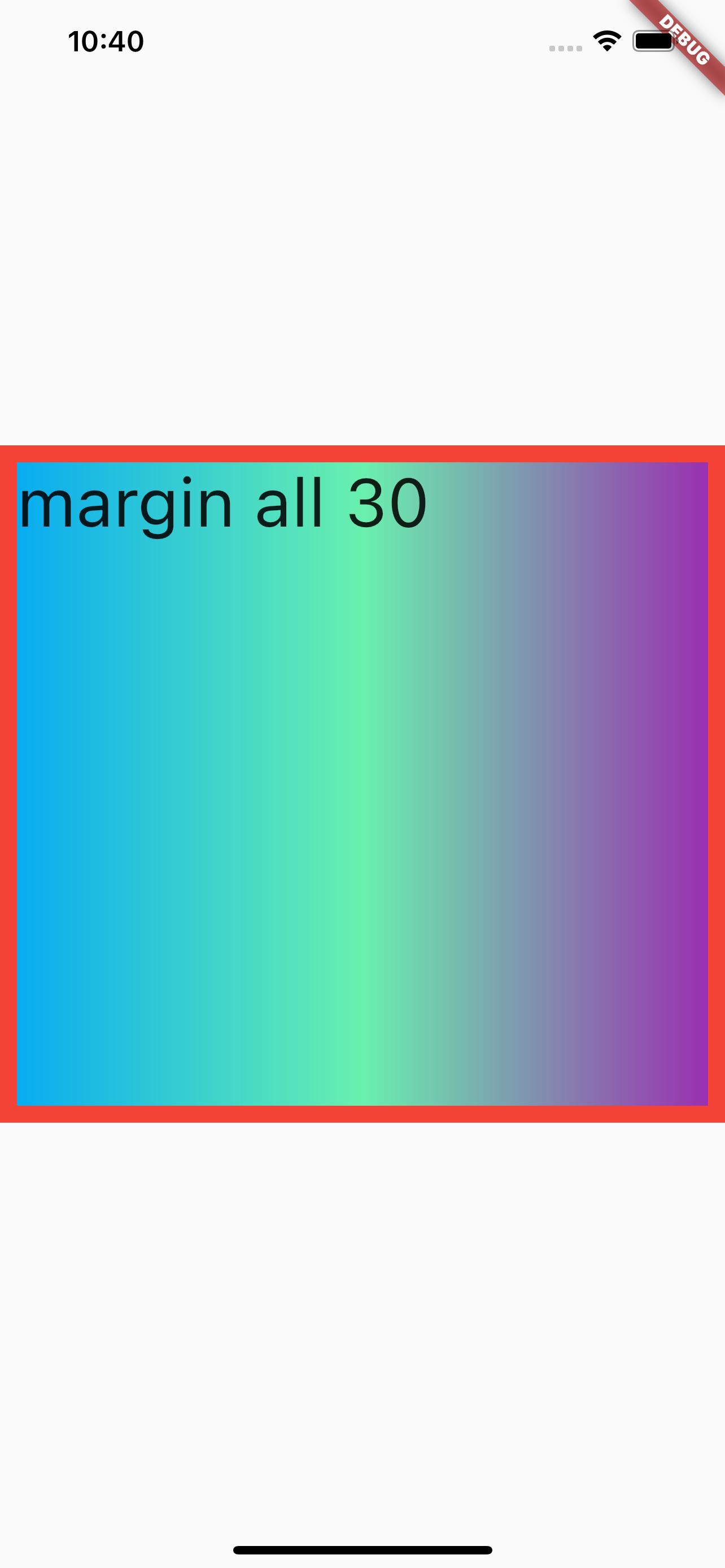
錯誤:#
The following assertion was thrown building MyApp(dirty):
Cannot provide both a color and a decoration
To provide both, use "decoration: BoxDecoration(color: color)".
'package/src/widgets/container.dart':
Failed assertion: line 274 pos 15: 'color == null || decoration == null'
錯誤代碼如下:
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Container Learn',
home: Scaffold(
body: Center(
child: Container(
child: Text(
'Container Text',
style: TextStyle(fontSize: 40.0),
),
alignment: Alignment.topLeft,
color: Colors.lightBlue,
padding: const EdgeInsets.fromLTRB(10.0, 30.0, 0.0, 0.0),
margin: const EdgeInsets.all(30.0),
decoration: BoxDecoration(
gradient: const LinearGradient(colors: [
Colors.lightBlue,
Colors.greenAccent,
Colors.purple,
]),
border: Border.all(width: 10.0, color: Colors.red)),
),
),
),
);
}
}
原因:Container 的 color 和 decoration 不能同時設定,如果需要設定這兩個,可以通過設定BoxDecoration(color: color)
來實現