Flutter Component Basics - Container#
Container is a container component, similar to the
Properties of Container#
Common properties of Container are as follows:
- Container
- child: Child view
- alignment: Alignment of the child view
- topLeft: Top left alignment
- topCenter: Top center alignment
- topRight: Top right alignment
- centerLeft: Center left alignment
- center: Center alignment
- centerRight: Center right alignment
- bottomLeft: Bottom left alignment
- bottomCenter: Bottom center alignment
- bottomRight: Bottom right alignment
- color: Background color
- width: Width
- height: Height
- padding: Margin of the child view from the Container
- margin: Margin of the Container from the parent view
- decoration: Decoration
Child View Alignment - alignment#
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Container Learn',
home: Scaffold(
body: Center(
child: Container(
child: Text(
'Alignment center',
style: TextStyle(fontSize: 40.0),
),
alignment: Alignment.center,
width: 500.0,
height: 400.0,
color: Colors.lightBlue,
),
),
),
);
}
}
The effect is as follows:
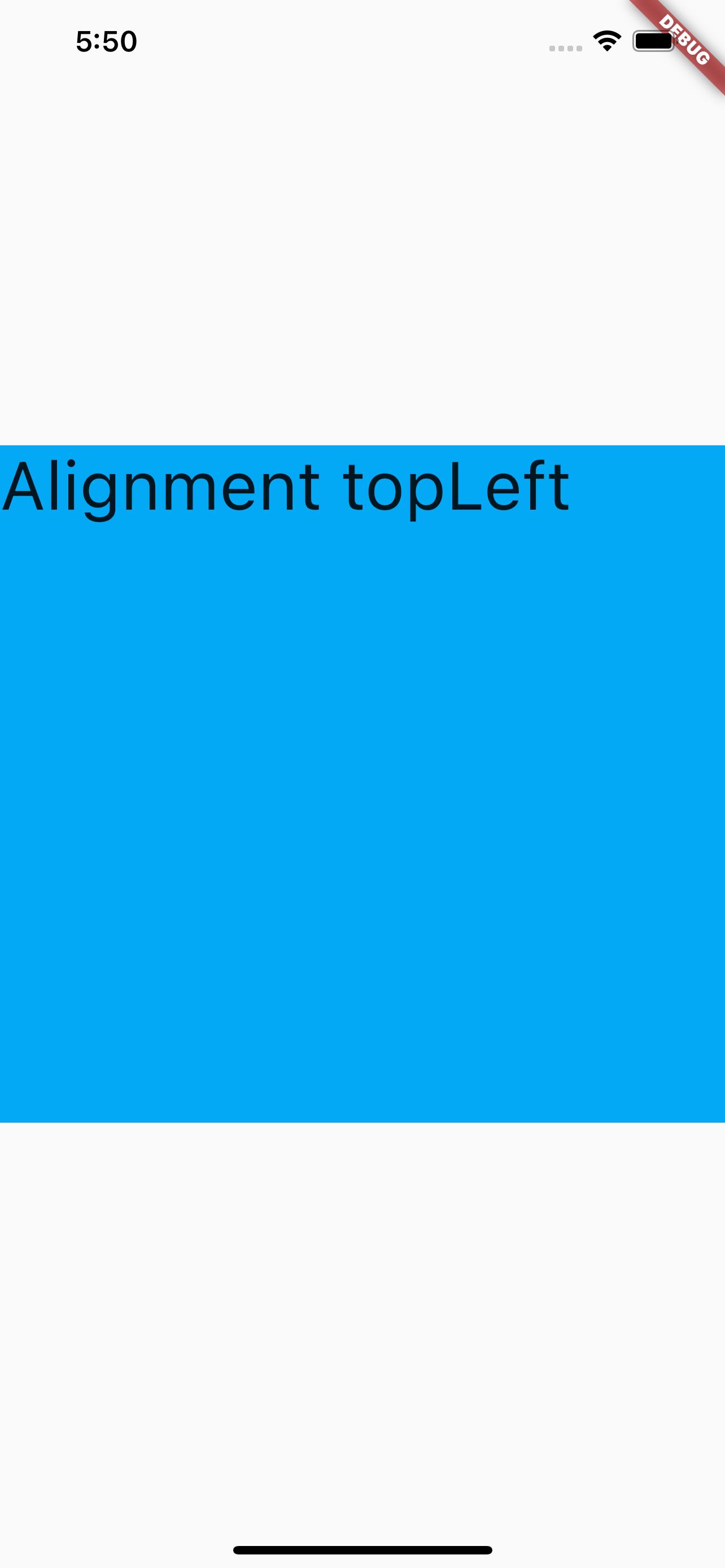
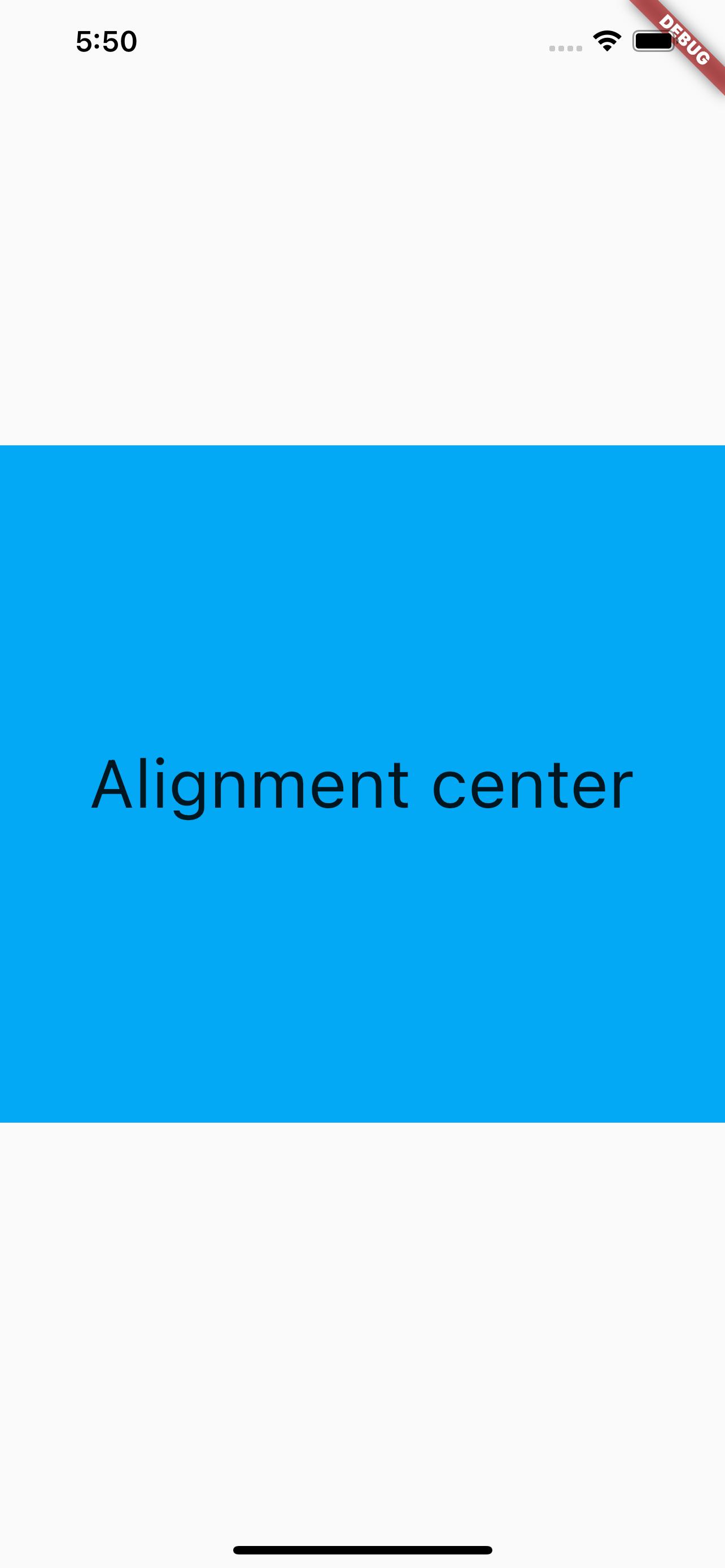
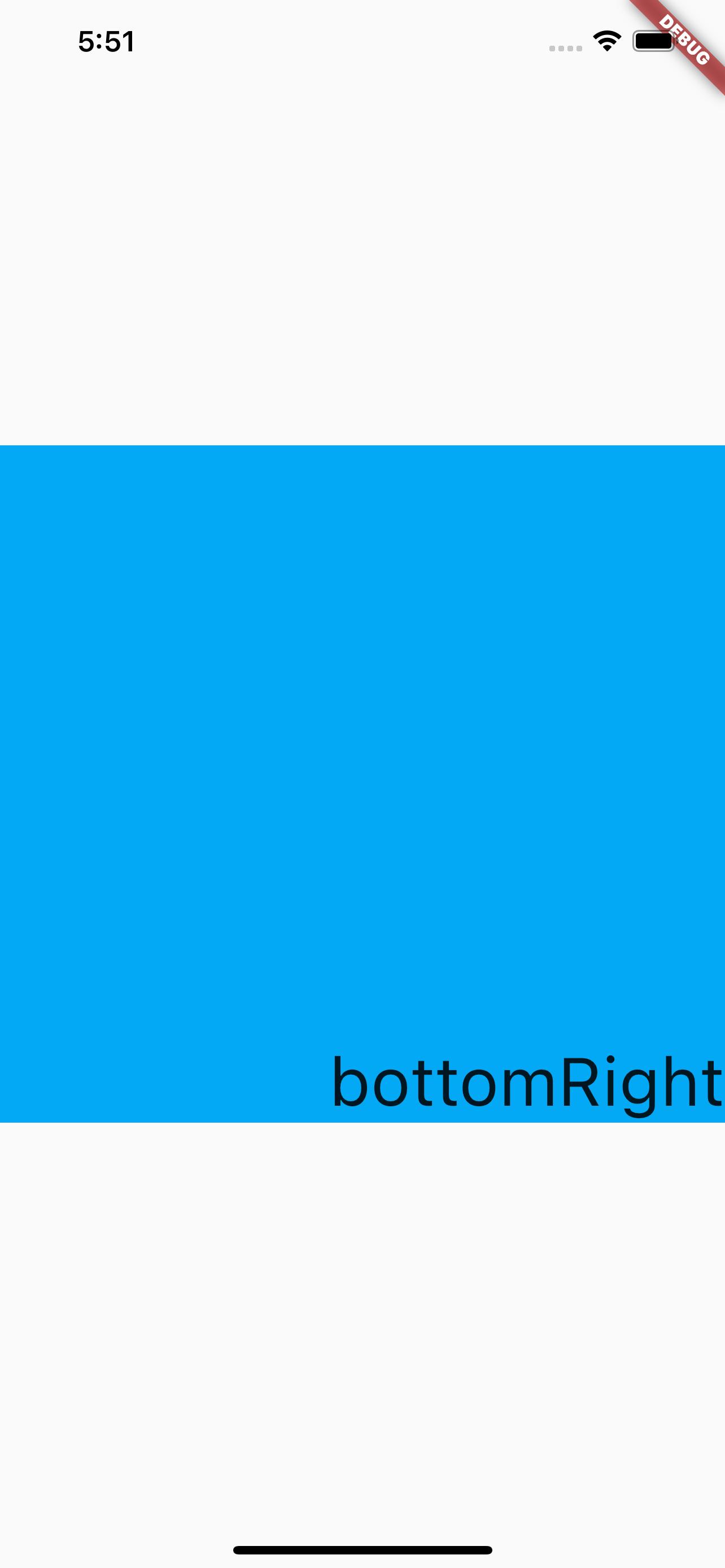
Container Width and Height#
The settings for width and height are fixed values.
There is no setting like '100%' as in H5. Therefore, if you want to set the Container to the screen width and height, you can use the following methods:
Method 1:
import 'dart:ui';
final width = window.physicalSize.width;
final height = window.physicalSize.height;
Container(
color: Colors.red,
width: width,
child: Text("How wide is the width"),
)
Method 2:
Container(
color: Colors.red,
width: double.infinity,
child: Text("How wide is the width"),
)
Padding - Margin of Child View from Container#
Padding sets the margin of the child view from the Container. There are usually two ways to set it: EdgeInsets.all
is commonly used to set all margins to be the same; EdgeInsets.fromLTRB
is used to set specific margins (LTRB corresponds to Left, Top, Right, Bottom). The code is as follows:
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Container Learn',
home: Scaffold(
body: Center(
child: Container(
child: Text(
'padding left: 10, top: 20',
style: TextStyle(fontSize: 40.0),
),
alignment: Alignment.topLeft,
width: 500.0,
height: 400.0,
color: Colors.lightBlue,
padding: const EdgeInsets.fromLTRB(10.0, 20.0, 0.0, 0.0),
// padding: const EdgeInsets.all(20),
),
),
),
);
}
}
The display effect is as follows:
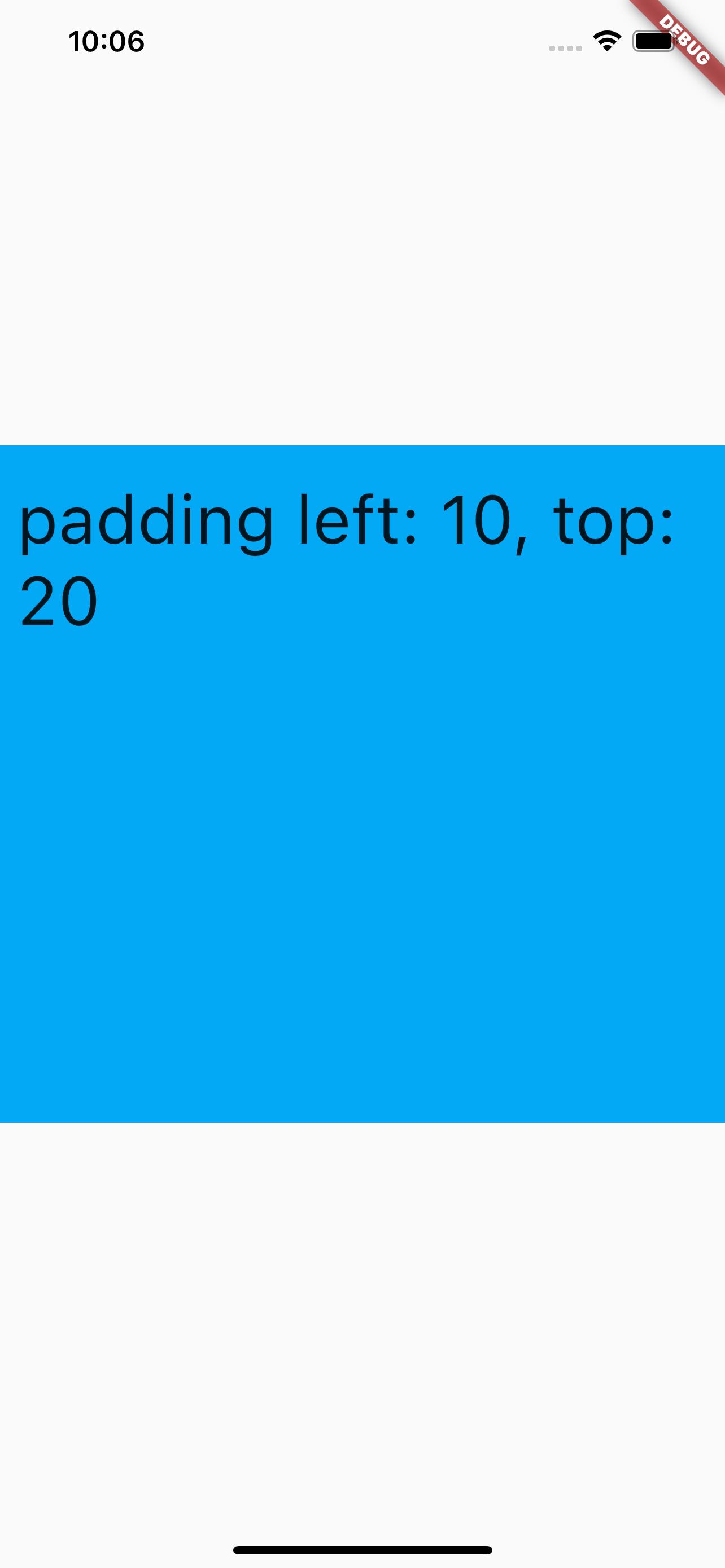
Margin - Margin of Container from Parent View#
The setting of margin is the same as padding. For a comparative effect, you can first comment out width and height. The code is as follows:
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Container Learn',
home: Scaffold(
body: Center(
child: Container(
child: Text(
'margin all 30',
style: TextStyle(fontSize: 40.0),
),
alignment: Alignment.topLeft,
// width: 500.0,
// height: 400.0,
color: Colors.lightBlue,
// padding: const EdgeInsets.fromLTRB(10.0, 20.0, 0.0, 0.0),
// padding: const EdgeInsets.all(20),
margin: const EdgeInsets.all(30.0),
),
),
),
);
}
}
The effect is as follows:
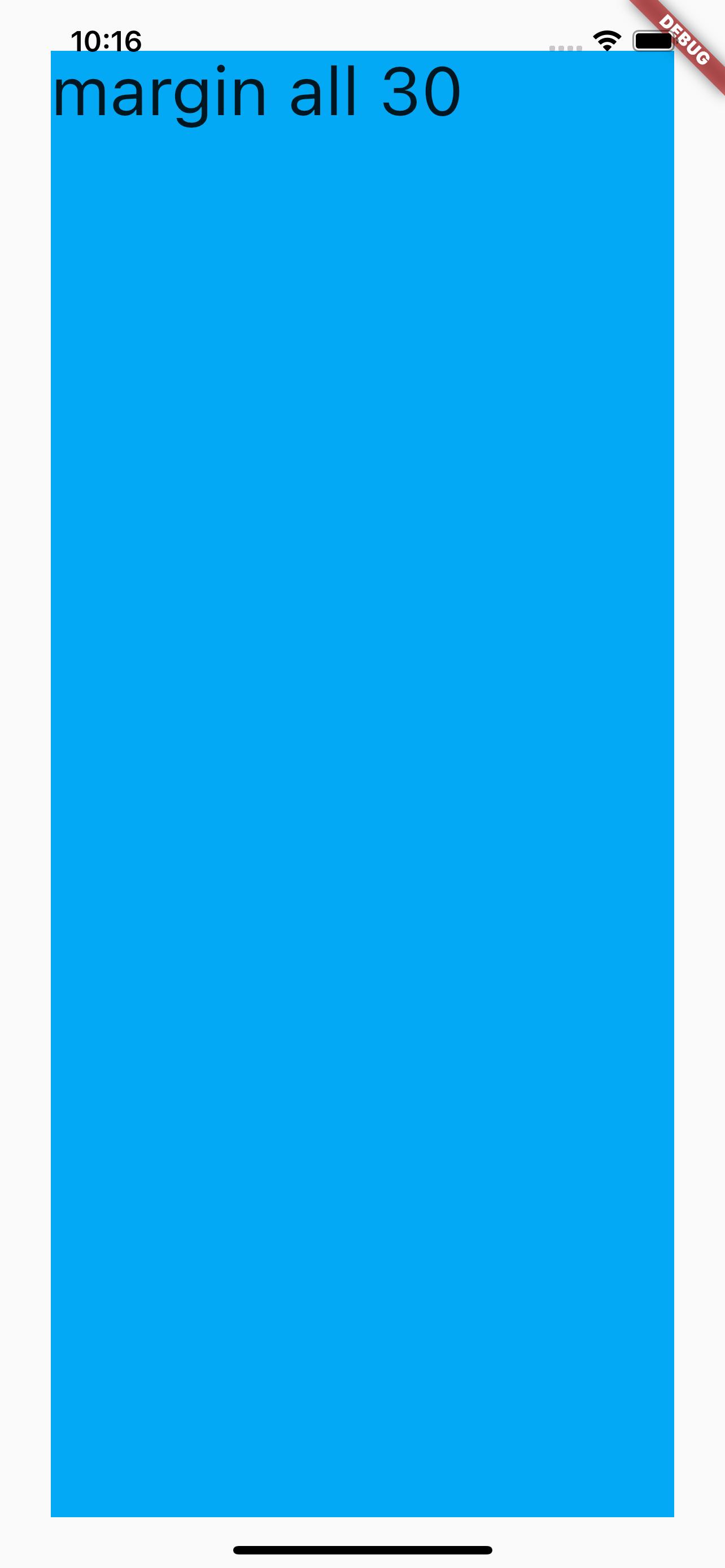
Decoration of Container#
Decoration can be used to set background color, background gradient effects, border effects, etc. It is important to note that decoration and color cannot be set simultaneously. If you need to set both, you can do so by setting color in the decoration. The code is as follows:
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Container Learn',
home: Scaffold(
body: Center(
child: Container(
child: Text(
'margin all 30',
style: TextStyle(fontSize: 40.0),
),
alignment: Alignment.topLeft,
width: 500.0,
height: 400,
// color: Colors.lightBlue,
// padding: const EdgeInsets.fromLTRB(10.0, 20.0, 0.0, 0.0),
// padding: const EdgeInsets.all(20),
// margin: const EdgeInsets.all(30.0),
decoration: BoxDecoration(
gradient: const LinearGradient(colors: [
Colors.lightBlue,
Colors.greenAccent,
Colors.purple,
]),
border: Border.all(width: 10.0, color: Colors.red),
color: Colors.lightBlue)),
),
),
);
}
}
The effect is as follows:
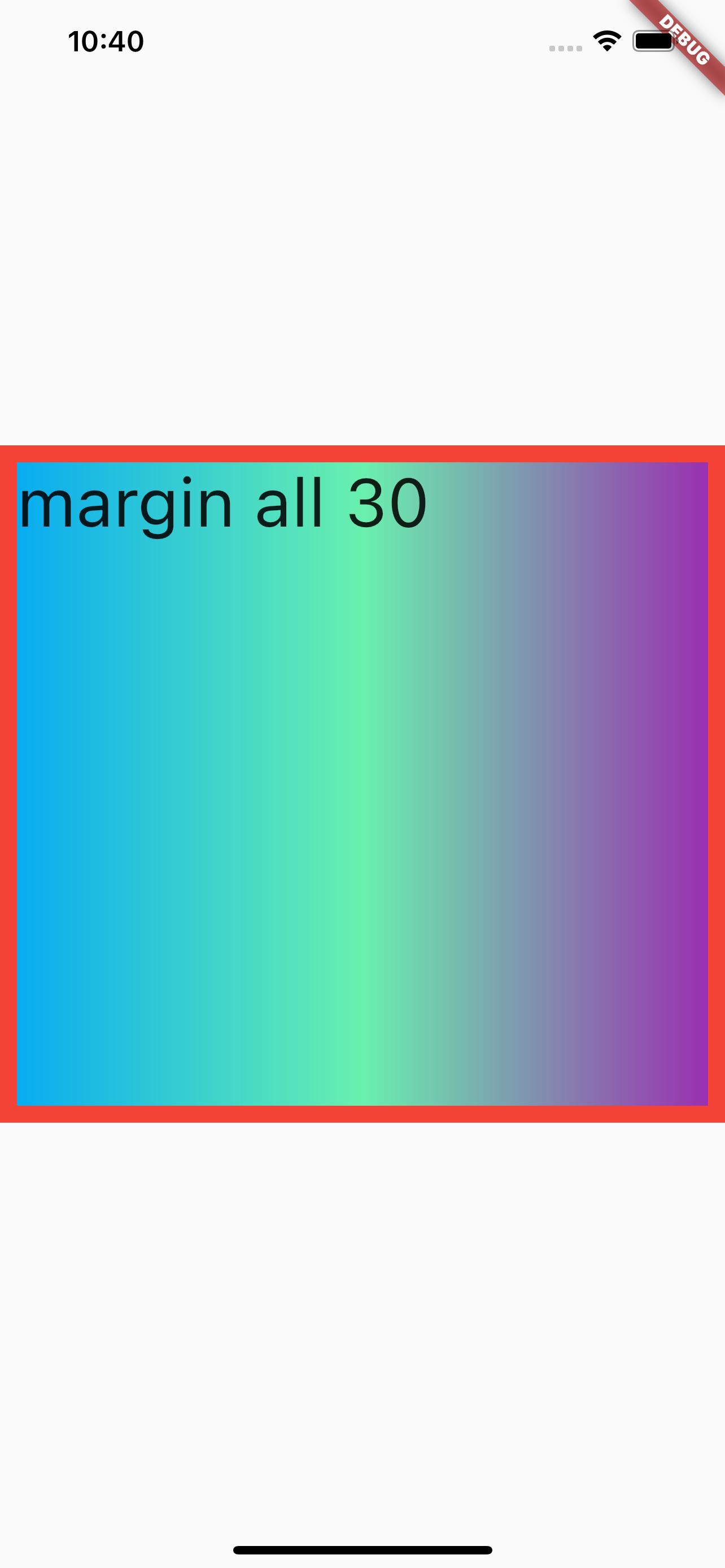
Error:#
The following assertion was thrown building MyApp(dirty):
Cannot provide both a color and a decoration
To provide both, use "decoration: BoxDecoration(color: color)".
'package/src/widgets/container.dart':
Failed assertion: line 274 pos 15: 'color == null || decoration == null'
The erroneous code is as follows:
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Container Learn',
home: Scaffold(
body: Center(
child: Container(
child: Text(
'Container Text',
style: TextStyle(fontSize: 40.0),
),
alignment: Alignment.topLeft,
color: Colors.lightBlue,
padding: const EdgeInsets.fromLTRB(10.0, 30.0, 0.0, 0.0),
margin: const EdgeInsets.all(30.0),
decoration: BoxDecoration(
gradient: const LinearGradient(colors: [
Colors.lightBlue,
Colors.greenAccent,
Colors.purple,
]),
border: Border.all(width: 10.0, color: Colors.red)),
),
),
),
);
}
}
Reason: The color and decoration of the Container cannot be set simultaneously. If both need to be set, you can achieve this by setting BoxDecoration(color: color)
.
References#
Flutter Container Doc
Flutter Free Video Season 2 - Common Components